The Telerik ComboBox for ASP.NET allows you to define an ItemTemplate which gives you the possibility to customize the appearance and functionality of the ComboBox. This article provides an example on how to place a multi-select TreeView inside an ItemTemplate, handling the selection on client side and how to process the data on the server side.
Telerik provides an example of placing a TreeView in a ComboBox here . But what if you want a multiple selection TreeView (with tri-state check boxes) ?
The ComboBox with the multi-select TreeView described in this article looks like this .
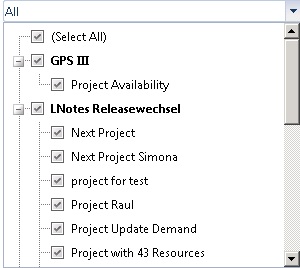
We are going to begin with the .aspx page and the Javascript that handles TreeView selection on the client side.
The code that needs to be placed inside the .aspx page :
<telerik:RadComboBox ID="rcbProject" runat="server" Width="100%" AllowCustomText="true" OnClientDropDownClosed="OnClientDropDownClosing" EmptyMessage="Select Project/Project Group" Skin="Windows7" DropDownWidth="300px" Height="300px" MaxHeight="400px" OnLoad="RcbProject_Load" > <ItemTemplate> <div id="div1" onclick="StopPropagation(event)"> <telerik:RadTreeView runat="server" ID="projectTree" CheckBoxes="True" CheckChildNodes="True" TriStateCheckBoxes="True" OnClientNodeChecked="nodeChecked" OnClientNodeClicked="nodeClicked"> <Nodes> <telerik:RadTreeNode runat="server" Text="(Select All)" Value="SelectAll" Expanded="false" /> </Nodes> </telerik:RadTreeView> </div> </ItemTemplate> <Items> <telerik:RadComboBoxItem Text="" Owner="rcbProject" /> </Items> </telerik:RadComboBox> |
As you can see from the code above, we’re not adding the TreeView directly to the ItemTemplate, instead we add a div with a click handler and we place the TreeView inside this div element. This is required to stop the drop down from closing when the user clicks on a node from the TreeView.
You can find an example and some explanations of the properties used in a TreeView with check boxes here.
We are going to focus on the nodeChecked and nodeCliked but before we look at those functions, note that we added a node to the tree to allow selecting/deselecting all the nodes at once, and also, in the Items collection of the ComboBox we have to add an item with no text (this is needed for the tree to be shown).
When a node is checked, the text displayed in that node will be added to the ComboBox Text property. This is achieved using the following Javascript code :
function nodeClicked(sender, args) { var node = args.get_node(); if (node.get_checked()) { node.uncheck(); } else { node.check(); } nodeChecked(sender, args) } function nodeChecked(sender, args) { var comboBox = $find(""); //check if 'Select All' node has been checked/unchecked var tempNode = args.get_node(); if (tempNode.get_text().toString() == "(Select All)") { // check or uncheck all the nodes } else { var nodes = new Array(); nodes = sender.get_checkedNodes(); var vals = ""; var i = 0; for (i = 0; i < nodes.length; i++) { var n = nodes[i]; var nodeText=n.get_text().toString(); if(nodeText!="(Select All)") { vals = vals + n.get_text().toString() + ","; } } //prevent combo from closing supressDropDownClosing = true; comboBox.set_text(vals); } } |
As you can see, when a node from the tree is clicked, we select/deselect the node and call the nodeChecked function. The ‘nodeChecked’ funtion checks if the node containing the “Select All” text has been clicked, if this is the case you can select or deselect all the nodes from the tree (this code has been omitted for simplicity).
If the user clicked another node we loop through the collection of checked nodes and store all the text displayed by this nodes in a local variable that will be used to set the ‘Text’ property of the ComboBox.
If you remember, when we declared the combo box , the TreeView was added inside a div element witch had a click handler associated. When the user performs a click on the div element (this means every time the user selects a node from the TreeView) the StopPropagation function that will cancel the event bubbling will be called.
function StopPropagation(e) { //cancel bubbling e.cancelBubble = true; if (e.stopPropagation) { e.stopPropagation(); } } |
Moving to the server side, you can bind the TreeView to a data source and you can also change the mode nodes are displayed. To bind a tree to a data source you need the following fields : DataFieldID , DataFieldParentID , DataTextField , DataValueField . The TreeView object can be accesed from the ComboBox by calling FindControl with the id of the tree on the first item of the combo box. Here’s an example of how you can do that :
// project combo DataTable projects = resourceDataSet.Tables[3]; RadTreeView prjTree = rcbProject.Items[0].FindControl("projectTree") as RadTreeView; prjTree.DataFieldID = "NodeID"; prjTree.DataFieldParentID = "ParentNodeID"; prjTree.DataTextField = "ProjectName"; prjTree.DataValueField = "ProjectUID"; prjTree.AppendDataBoundItems = true; prjTree.DataSource = projects; prjTree.DataBind(); |
If you want to add a node on the first level (with no parents) the DataFieldParentID field must be empty .
In the image from the beginning of the article you can see that all the nodes are checked and the nodes that have children are displayed with bold font. All that is done on the combo box Load event and here is the code :
protected void RcbProject_Load(object sender, EventArgs e) { RadTreeView prjTree = rcbProject.Items[0].FindControl("projectTree") as RadTreeView; if (!this.IsWebPartPostBack) { foreach (RadTreeNode itm in prjTree.Nodes) { itm.Checked = true; if (itm.Nodes.Count > 0) { itm.Font.Bold = true; // perform this check on child nodes } } this.rcbProject.Text = "All"; } } |
To get the value of a checked node you can do the following :
private string GetSelectedIds() { RadTreeView theTree = this.rcbProject.Items[0].FindControl("projectTree") as RadTreeView; List nodes = theTree.CheckedNodes.ToList(); foreach (RadTreeNode nd in nodes) { strBuilder.Append(nd.Value.ToString()); strBuilder.Append(","); } return value.Length > 0 ? value.Substring(0, value.Length - 1) : string.Empty; } |
The above method returns a string with the ids of the selected nodes (the value that is bound to the DataValueField property) separated by a comma. You could also get the text displayed in the node using :
string test=nd.Text; |
That’s it, hope you find this useful.
Finally, there’s another very important peculiarity of what does Cialis that brings it so high above its alternatives. It is the only med that is available in two versions – one intended for use on as-needed basis and one intended for daily use. As you might know, Viagra and Levitra only come in the latter of these two forms and should be consumed shortly before expected sexual activity to ensure best effect. Daily Cialis, in its turn, contains low doses of Tadalafil, which allows to build its concentration up in your system gradually over time and maintain it on acceptable levels, which, consequently, makes it possible for you to enjoy sex at any moment without having to time it.
Can you please tell how to use it JSP page ?
Thanks
January 6, 2012 at 9:10 amOnClientDropDownClosed=”OnClientDropDownClosing” == event is creating problem
January 6, 2012 at 3:02 pmgood content
September 11, 2012 at 2:25 am